datarobot.NotificationChannel
Explore with Pulumi AI
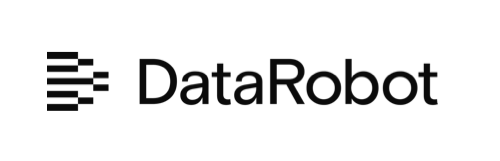
Notification Channel
Example Usage
import * as pulumi from "@pulumi/pulumi";
import * as datarobot from "@datarobot/pulumi-datarobot";
const example = new datarobot.NotificationChannel("example", {
channelType: "DataRobotUser",
relatedEntityId: datarobot_deployment.example.id,
relatedEntityType: "deployment",
contentType: "application/json",
customHeaders: [{
name: "header1",
value: "value1",
}],
drEntities: [{
id: "11111111111111",
name: "example user",
}],
languageCode: "en",
emailAddress: "example@datarobot.com",
payloadUrl: "https://example.com",
secretToken: "example_secret_token",
validateSsl: true,
verificationCode: "11111",
});
export const datarobotNotificationPolicyId = datarobot_notification_policy.example.id;
import pulumi
import pulumi_datarobot as datarobot
example = datarobot.NotificationChannel("example",
channel_type="DataRobotUser",
related_entity_id=datarobot_deployment["example"]["id"],
related_entity_type="deployment",
content_type="application/json",
custom_headers=[{
"name": "header1",
"value": "value1",
}],
dr_entities=[{
"id": "11111111111111",
"name": "example user",
}],
language_code="en",
email_address="example@datarobot.com",
payload_url="https://example.com",
secret_token="example_secret_token",
validate_ssl=True,
verification_code="11111")
pulumi.export("datarobotNotificationPolicyId", datarobot_notification_policy["example"]["id"])
package main
import (
"github.com/datarobot-community/pulumi-datarobot/sdk/go/datarobot"
"github.com/pulumi/pulumi/sdk/v3/go/pulumi"
)
func main() {
pulumi.Run(func(ctx *pulumi.Context) error {
_, err := datarobot.NewNotificationChannel(ctx, "example", &datarobot.NotificationChannelArgs{
ChannelType: pulumi.String("DataRobotUser"),
RelatedEntityId: pulumi.Any(datarobot_deployment.Example.Id),
RelatedEntityType: pulumi.String("deployment"),
ContentType: pulumi.String("application/json"),
CustomHeaders: datarobot.NotificationChannelCustomHeaderArray{
&datarobot.NotificationChannelCustomHeaderArgs{
Name: pulumi.String("header1"),
Value: pulumi.String("value1"),
},
},
DrEntities: datarobot.NotificationChannelDrEntityArray{
&datarobot.NotificationChannelDrEntityArgs{
Id: pulumi.String("11111111111111"),
Name: pulumi.String("example user"),
},
},
LanguageCode: pulumi.String("en"),
EmailAddress: pulumi.String("example@datarobot.com"),
PayloadUrl: pulumi.String("https://example.com"),
SecretToken: pulumi.String("example_secret_token"),
ValidateSsl: pulumi.Bool(true),
VerificationCode: pulumi.String("11111"),
})
if err != nil {
return err
}
ctx.Export("datarobotNotificationPolicyId", datarobot_notification_policy.Example.Id)
return nil
})
}
using System.Collections.Generic;
using System.Linq;
using Pulumi;
using Datarobot = DataRobotPulumi.Datarobot;
return await Deployment.RunAsync(() =>
{
var example = new Datarobot.NotificationChannel("example", new()
{
ChannelType = "DataRobotUser",
RelatedEntityId = datarobot_deployment.Example.Id,
RelatedEntityType = "deployment",
ContentType = "application/json",
CustomHeaders = new[]
{
new Datarobot.Inputs.NotificationChannelCustomHeaderArgs
{
Name = "header1",
Value = "value1",
},
},
DrEntities = new[]
{
new Datarobot.Inputs.NotificationChannelDrEntityArgs
{
Id = "11111111111111",
Name = "example user",
},
},
LanguageCode = "en",
EmailAddress = "example@datarobot.com",
PayloadUrl = "https://example.com",
SecretToken = "example_secret_token",
ValidateSsl = true,
VerificationCode = "11111",
});
return new Dictionary<string, object?>
{
["datarobotNotificationPolicyId"] = datarobot_notification_policy.Example.Id,
};
});
package generated_program;
import com.pulumi.Context;
import com.pulumi.Pulumi;
import com.pulumi.core.Output;
import com.pulumi.datarobot.NotificationChannel;
import com.pulumi.datarobot.NotificationChannelArgs;
import com.pulumi.datarobot.inputs.NotificationChannelCustomHeaderArgs;
import com.pulumi.datarobot.inputs.NotificationChannelDrEntityArgs;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
public class App {
public static void main(String[] args) {
Pulumi.run(App::stack);
}
public static void stack(Context ctx) {
var example = new NotificationChannel("example", NotificationChannelArgs.builder()
.channelType("DataRobotUser")
.relatedEntityId(datarobot_deployment.example().id())
.relatedEntityType("deployment")
.contentType("application/json")
.customHeaders(NotificationChannelCustomHeaderArgs.builder()
.name("header1")
.value("value1")
.build())
.drEntities(NotificationChannelDrEntityArgs.builder()
.id("11111111111111")
.name("example user")
.build())
.languageCode("en")
.emailAddress("example@datarobot.com")
.payloadUrl("https://example.com")
.secretToken("example_secret_token")
.validateSsl(true)
.verificationCode("11111")
.build());
ctx.export("datarobotNotificationPolicyId", datarobot_notification_policy.example().id());
}
}
resources:
example:
type: datarobot:NotificationChannel
properties:
channelType: DataRobotUser
relatedEntityId: ${datarobot_deployment.example.id}
relatedEntityType: deployment
# Optional
contentType: application/json
customHeaders:
- name: header1
value: value1
drEntities:
- id: '11111111111111'
name: example user
languageCode: en
emailAddress: example@datarobot.com
payloadUrl: https://example.com
secretToken: example_secret_token
validateSsl: true
verificationCode: '11111'
outputs:
datarobotNotificationPolicyId: ${datarobot_notification_policy.example.id}
Create NotificationChannel Resource
Resources are created with functions called constructors. To learn more about declaring and configuring resources, see Resources.
Constructor syntax
new NotificationChannel(name: string, args: NotificationChannelArgs, opts?: CustomResourceOptions);
@overload
def NotificationChannel(resource_name: str,
args: NotificationChannelArgs,
opts: Optional[ResourceOptions] = None)
@overload
def NotificationChannel(resource_name: str,
opts: Optional[ResourceOptions] = None,
channel_type: Optional[str] = None,
related_entity_type: Optional[str] = None,
related_entity_id: Optional[str] = None,
dr_entities: Optional[Sequence[NotificationChannelDrEntityArgs]] = None,
email_address: Optional[str] = None,
language_code: Optional[str] = None,
name: Optional[str] = None,
payload_url: Optional[str] = None,
custom_headers: Optional[Sequence[NotificationChannelCustomHeaderArgs]] = None,
content_type: Optional[str] = None,
secret_token: Optional[str] = None,
validate_ssl: Optional[bool] = None,
verification_code: Optional[str] = None)
func NewNotificationChannel(ctx *Context, name string, args NotificationChannelArgs, opts ...ResourceOption) (*NotificationChannel, error)
public NotificationChannel(string name, NotificationChannelArgs args, CustomResourceOptions? opts = null)
public NotificationChannel(String name, NotificationChannelArgs args)
public NotificationChannel(String name, NotificationChannelArgs args, CustomResourceOptions options)
type: datarobot:NotificationChannel
properties: # The arguments to resource properties.
options: # Bag of options to control resource's behavior.
Parameters
- name string
- The unique name of the resource.
- args NotificationChannelArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- resource_name str
- The unique name of the resource.
- args NotificationChannelArgs
- The arguments to resource properties.
- opts ResourceOptions
- Bag of options to control resource's behavior.
- ctx Context
- Context object for the current deployment.
- name string
- The unique name of the resource.
- args NotificationChannelArgs
- The arguments to resource properties.
- opts ResourceOption
- Bag of options to control resource's behavior.
- name string
- The unique name of the resource.
- args NotificationChannelArgs
- The arguments to resource properties.
- opts CustomResourceOptions
- Bag of options to control resource's behavior.
- name String
- The unique name of the resource.
- args NotificationChannelArgs
- The arguments to resource properties.
- options CustomResourceOptions
- Bag of options to control resource's behavior.
Constructor example
The following reference example uses placeholder values for all input properties.
var notificationChannelResource = new Datarobot.NotificationChannel("notificationChannelResource", new()
{
ChannelType = "string",
RelatedEntityType = "string",
RelatedEntityId = "string",
DrEntities = new[]
{
new Datarobot.Inputs.NotificationChannelDrEntityArgs
{
Id = "string",
Name = "string",
},
},
EmailAddress = "string",
LanguageCode = "string",
Name = "string",
PayloadUrl = "string",
CustomHeaders = new[]
{
new Datarobot.Inputs.NotificationChannelCustomHeaderArgs
{
Name = "string",
Value = "string",
},
},
ContentType = "string",
SecretToken = "string",
ValidateSsl = false,
VerificationCode = "string",
});
example, err := datarobot.NewNotificationChannel(ctx, "notificationChannelResource", &datarobot.NotificationChannelArgs{
ChannelType: pulumi.String("string"),
RelatedEntityType: pulumi.String("string"),
RelatedEntityId: pulumi.String("string"),
DrEntities: datarobot.NotificationChannelDrEntityArray{
&datarobot.NotificationChannelDrEntityArgs{
Id: pulumi.String("string"),
Name: pulumi.String("string"),
},
},
EmailAddress: pulumi.String("string"),
LanguageCode: pulumi.String("string"),
Name: pulumi.String("string"),
PayloadUrl: pulumi.String("string"),
CustomHeaders: datarobot.NotificationChannelCustomHeaderArray{
&datarobot.NotificationChannelCustomHeaderArgs{
Name: pulumi.String("string"),
Value: pulumi.String("string"),
},
},
ContentType: pulumi.String("string"),
SecretToken: pulumi.String("string"),
ValidateSsl: pulumi.Bool(false),
VerificationCode: pulumi.String("string"),
})
var notificationChannelResource = new NotificationChannel("notificationChannelResource", NotificationChannelArgs.builder()
.channelType("string")
.relatedEntityType("string")
.relatedEntityId("string")
.drEntities(NotificationChannelDrEntityArgs.builder()
.id("string")
.name("string")
.build())
.emailAddress("string")
.languageCode("string")
.name("string")
.payloadUrl("string")
.customHeaders(NotificationChannelCustomHeaderArgs.builder()
.name("string")
.value("string")
.build())
.contentType("string")
.secretToken("string")
.validateSsl(false)
.verificationCode("string")
.build());
notification_channel_resource = datarobot.NotificationChannel("notificationChannelResource",
channel_type="string",
related_entity_type="string",
related_entity_id="string",
dr_entities=[{
"id": "string",
"name": "string",
}],
email_address="string",
language_code="string",
name="string",
payload_url="string",
custom_headers=[{
"name": "string",
"value": "string",
}],
content_type="string",
secret_token="string",
validate_ssl=False,
verification_code="string")
const notificationChannelResource = new datarobot.NotificationChannel("notificationChannelResource", {
channelType: "string",
relatedEntityType: "string",
relatedEntityId: "string",
drEntities: [{
id: "string",
name: "string",
}],
emailAddress: "string",
languageCode: "string",
name: "string",
payloadUrl: "string",
customHeaders: [{
name: "string",
value: "string",
}],
contentType: "string",
secretToken: "string",
validateSsl: false,
verificationCode: "string",
});
type: datarobot:NotificationChannel
properties:
channelType: string
contentType: string
customHeaders:
- name: string
value: string
drEntities:
- id: string
name: string
emailAddress: string
languageCode: string
name: string
payloadUrl: string
relatedEntityId: string
relatedEntityType: string
secretToken: string
validateSsl: false
verificationCode: string
NotificationChannel Resource Properties
To learn more about resource properties and how to use them, see Inputs and Outputs in the Architecture and Concepts docs.
Inputs
In Python, inputs that are objects can be passed either as argument classes or as dictionary literals.
The NotificationChannel resource accepts the following input properties:
- Channel
Type string - The Type of Notification Channel.
- string
- The ID of related entity.
- string
- The type of related entity.
- Content
Type string - The content type of the messages of the Notification Channel.
- Custom
Headers List<DataRobot Notification Channel Custom Header> - Custom headers and their values to be sent in the Notification Channel.
- Dr
Entities List<DataRobot Notification Channel Dr Entity> - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- Email
Address string - The email address to be used in the Notification Channel.
- Language
Code string - The preferred language code.
- Name string
- The name of the Notification Channel.
- Payload
Url string - The payload URL of the Notification Channel.
- Secret
Token string - The secret token to be used for the Notification Channel.
- Validate
Ssl bool - Defines if validate ssl or not in the Notification Channel.
- Verification
Code string - Required if the channel type is email.
- Channel
Type string - The Type of Notification Channel.
- string
- The ID of related entity.
- string
- The type of related entity.
- Content
Type string - The content type of the messages of the Notification Channel.
- Custom
Headers []NotificationChannel Custom Header Args - Custom headers and their values to be sent in the Notification Channel.
- Dr
Entities []NotificationChannel Dr Entity Args - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- Email
Address string - The email address to be used in the Notification Channel.
- Language
Code string - The preferred language code.
- Name string
- The name of the Notification Channel.
- Payload
Url string - The payload URL of the Notification Channel.
- Secret
Token string - The secret token to be used for the Notification Channel.
- Validate
Ssl bool - Defines if validate ssl or not in the Notification Channel.
- Verification
Code string - Required if the channel type is email.
- channel
Type String - The Type of Notification Channel.
- String
- The ID of related entity.
- String
- The type of related entity.
- content
Type String - The content type of the messages of the Notification Channel.
- custom
Headers List<NotificationChannel Custom Header> - Custom headers and their values to be sent in the Notification Channel.
- dr
Entities List<NotificationChannel Dr Entity> - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email
Address String - The email address to be used in the Notification Channel.
- language
Code String - The preferred language code.
- name String
- The name of the Notification Channel.
- payload
Url String - The payload URL of the Notification Channel.
- secret
Token String - The secret token to be used for the Notification Channel.
- validate
Ssl Boolean - Defines if validate ssl or not in the Notification Channel.
- verification
Code String - Required if the channel type is email.
- channel
Type string - The Type of Notification Channel.
- string
- The ID of related entity.
- string
- The type of related entity.
- content
Type string - The content type of the messages of the Notification Channel.
- custom
Headers NotificationChannel Custom Header[] - Custom headers and their values to be sent in the Notification Channel.
- dr
Entities NotificationChannel Dr Entity[] - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email
Address string - The email address to be used in the Notification Channel.
- language
Code string - The preferred language code.
- name string
- The name of the Notification Channel.
- payload
Url string - The payload URL of the Notification Channel.
- secret
Token string - The secret token to be used for the Notification Channel.
- validate
Ssl boolean - Defines if validate ssl or not in the Notification Channel.
- verification
Code string - Required if the channel type is email.
- channel_
type str - The Type of Notification Channel.
- str
- The ID of related entity.
- str
- The type of related entity.
- content_
type str - The content type of the messages of the Notification Channel.
- custom_
headers Sequence[NotificationChannel Custom Header Args] - Custom headers and their values to be sent in the Notification Channel.
- dr_
entities Sequence[NotificationChannel Dr Entity Args] - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email_
address str - The email address to be used in the Notification Channel.
- language_
code str - The preferred language code.
- name str
- The name of the Notification Channel.
- payload_
url str - The payload URL of the Notification Channel.
- secret_
token str - The secret token to be used for the Notification Channel.
- validate_
ssl bool - Defines if validate ssl or not in the Notification Channel.
- verification_
code str - Required if the channel type is email.
- channel
Type String - The Type of Notification Channel.
- String
- The ID of related entity.
- String
- The type of related entity.
- content
Type String - The content type of the messages of the Notification Channel.
- custom
Headers List<Property Map> - Custom headers and their values to be sent in the Notification Channel.
- dr
Entities List<Property Map> - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email
Address String - The email address to be used in the Notification Channel.
- language
Code String - The preferred language code.
- name String
- The name of the Notification Channel.
- payload
Url String - The payload URL of the Notification Channel.
- secret
Token String - The secret token to be used for the Notification Channel.
- validate
Ssl Boolean - Defines if validate ssl or not in the Notification Channel.
- verification
Code String - Required if the channel type is email.
Outputs
All input properties are implicitly available as output properties. Additionally, the NotificationChannel resource produces the following output properties:
- Id string
- The provider-assigned unique ID for this managed resource.
- Id string
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
- id string
- The provider-assigned unique ID for this managed resource.
- id str
- The provider-assigned unique ID for this managed resource.
- id String
- The provider-assigned unique ID for this managed resource.
Look up Existing NotificationChannel Resource
Get an existing NotificationChannel resource’s state with the given name, ID, and optional extra properties used to qualify the lookup.
public static get(name: string, id: Input<ID>, state?: NotificationChannelState, opts?: CustomResourceOptions): NotificationChannel
@staticmethod
def get(resource_name: str,
id: str,
opts: Optional[ResourceOptions] = None,
channel_type: Optional[str] = None,
content_type: Optional[str] = None,
custom_headers: Optional[Sequence[NotificationChannelCustomHeaderArgs]] = None,
dr_entities: Optional[Sequence[NotificationChannelDrEntityArgs]] = None,
email_address: Optional[str] = None,
language_code: Optional[str] = None,
name: Optional[str] = None,
payload_url: Optional[str] = None,
related_entity_id: Optional[str] = None,
related_entity_type: Optional[str] = None,
secret_token: Optional[str] = None,
validate_ssl: Optional[bool] = None,
verification_code: Optional[str] = None) -> NotificationChannel
func GetNotificationChannel(ctx *Context, name string, id IDInput, state *NotificationChannelState, opts ...ResourceOption) (*NotificationChannel, error)
public static NotificationChannel Get(string name, Input<string> id, NotificationChannelState? state, CustomResourceOptions? opts = null)
public static NotificationChannel get(String name, Output<String> id, NotificationChannelState state, CustomResourceOptions options)
resources: _: type: datarobot:NotificationChannel get: id: ${id}
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- resource_name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- name
- The unique name of the resulting resource.
- id
- The unique provider ID of the resource to lookup.
- state
- Any extra arguments used during the lookup.
- opts
- A bag of options that control this resource's behavior.
- Channel
Type string - The Type of Notification Channel.
- Content
Type string - The content type of the messages of the Notification Channel.
- Custom
Headers List<DataRobot Notification Channel Custom Header> - Custom headers and their values to be sent in the Notification Channel.
- Dr
Entities List<DataRobot Notification Channel Dr Entity> - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- Email
Address string - The email address to be used in the Notification Channel.
- Language
Code string - The preferred language code.
- Name string
- The name of the Notification Channel.
- Payload
Url string - The payload URL of the Notification Channel.
- string
- The ID of related entity.
- string
- The type of related entity.
- Secret
Token string - The secret token to be used for the Notification Channel.
- Validate
Ssl bool - Defines if validate ssl or not in the Notification Channel.
- Verification
Code string - Required if the channel type is email.
- Channel
Type string - The Type of Notification Channel.
- Content
Type string - The content type of the messages of the Notification Channel.
- Custom
Headers []NotificationChannel Custom Header Args - Custom headers and their values to be sent in the Notification Channel.
- Dr
Entities []NotificationChannel Dr Entity Args - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- Email
Address string - The email address to be used in the Notification Channel.
- Language
Code string - The preferred language code.
- Name string
- The name of the Notification Channel.
- Payload
Url string - The payload URL of the Notification Channel.
- string
- The ID of related entity.
- string
- The type of related entity.
- Secret
Token string - The secret token to be used for the Notification Channel.
- Validate
Ssl bool - Defines if validate ssl or not in the Notification Channel.
- Verification
Code string - Required if the channel type is email.
- channel
Type String - The Type of Notification Channel.
- content
Type String - The content type of the messages of the Notification Channel.
- custom
Headers List<NotificationChannel Custom Header> - Custom headers and their values to be sent in the Notification Channel.
- dr
Entities List<NotificationChannel Dr Entity> - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email
Address String - The email address to be used in the Notification Channel.
- language
Code String - The preferred language code.
- name String
- The name of the Notification Channel.
- payload
Url String - The payload URL of the Notification Channel.
- String
- The ID of related entity.
- String
- The type of related entity.
- secret
Token String - The secret token to be used for the Notification Channel.
- validate
Ssl Boolean - Defines if validate ssl or not in the Notification Channel.
- verification
Code String - Required if the channel type is email.
- channel
Type string - The Type of Notification Channel.
- content
Type string - The content type of the messages of the Notification Channel.
- custom
Headers NotificationChannel Custom Header[] - Custom headers and their values to be sent in the Notification Channel.
- dr
Entities NotificationChannel Dr Entity[] - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email
Address string - The email address to be used in the Notification Channel.
- language
Code string - The preferred language code.
- name string
- The name of the Notification Channel.
- payload
Url string - The payload URL of the Notification Channel.
- string
- The ID of related entity.
- string
- The type of related entity.
- secret
Token string - The secret token to be used for the Notification Channel.
- validate
Ssl boolean - Defines if validate ssl or not in the Notification Channel.
- verification
Code string - Required if the channel type is email.
- channel_
type str - The Type of Notification Channel.
- content_
type str - The content type of the messages of the Notification Channel.
- custom_
headers Sequence[NotificationChannel Custom Header Args] - Custom headers and their values to be sent in the Notification Channel.
- dr_
entities Sequence[NotificationChannel Dr Entity Args] - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email_
address str - The email address to be used in the Notification Channel.
- language_
code str - The preferred language code.
- name str
- The name of the Notification Channel.
- payload_
url str - The payload URL of the Notification Channel.
- str
- The ID of related entity.
- str
- The type of related entity.
- secret_
token str - The secret token to be used for the Notification Channel.
- validate_
ssl bool - Defines if validate ssl or not in the Notification Channel.
- verification_
code str - Required if the channel type is email.
- channel
Type String - The Type of Notification Channel.
- content
Type String - The content type of the messages of the Notification Channel.
- custom
Headers List<Property Map> - Custom headers and their values to be sent in the Notification Channel.
- dr
Entities List<Property Map> - The IDs of the DataRobot Users, Group or Custom Job associated with the DataRobotUser, DataRobotGroup or DataRobotCustomJob channel types.
- email
Address String - The email address to be used in the Notification Channel.
- language
Code String - The preferred language code.
- name String
- The name of the Notification Channel.
- payload
Url String - The payload URL of the Notification Channel.
- String
- The ID of related entity.
- String
- The type of related entity.
- secret
Token String - The secret token to be used for the Notification Channel.
- validate
Ssl Boolean - Defines if validate ssl or not in the Notification Channel.
- verification
Code String - Required if the channel type is email.
Supporting Types
NotificationChannelCustomHeader, NotificationChannelCustomHeaderArgs
NotificationChannelDrEntity, NotificationChannelDrEntityArgs
Package Details
- Repository
- datarobot datarobot-community/pulumi-datarobot
- License
- Apache-2.0
- Notes
- This Pulumi package is based on the
datarobot
Terraform Provider.
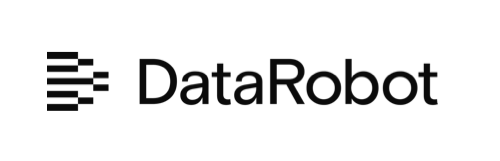